Using Amazon S3 with CakePHP
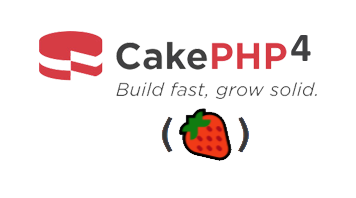
This describes how to install the S3 SDK with composer, and utilize S3
Install the AWS SDK in CakePHP:
From the folder of your project (e.g. c:\www\your-site), run the following command to install the AWS SDK into /vendor/aws/* and make available in your CakePHP4+ app:
composer require aws/aws-sdk-php
Next, you can use the S3 client as follows:
Set Up Your Client:
$s3Client = new S3Client( [ 'region' => 'ur-region-name', 'version' => 'latest, 'credentials' => [ 'key' => 'key', 'secret' => 'secret', ] ]);
Make a Call to S3:
// List the objects in the bucket $objects = $s3Client->listObjects([ 'Bucket' => 'bucket-name' ]); // Print the object keys if(isset($objects['Contents'])) { foreach ($objects['Contents'] as $object) { $s3files[] = $object; } return $s3files; } else { //debug('no objects in ' . $aws['bucket'] . ' bucket'); return false; }
Other Resources: