CakePHP 4 Component for Setting and Getting Cookies
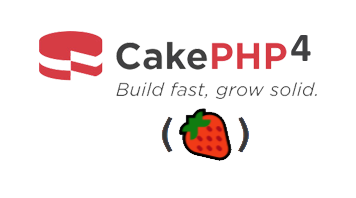
CakePHP 4 Component for Setting and Getting Cookies
This tutorial shows you how to create a component that sets a cookie on the user's browser. In this example, we use simple PHP instead of the Cake infrastructure for setting cookies and other values, so you can easily adapt this code to core PHP..
Step 1: Install the component
Create the file \src\Controller\Component\cookieComponent.php and paste the following code:
<?php namespace App\Controller\Component; use App\Controller\AppController; use Cake\Controller\Component; use Cake\Controller\ComponentRegistry; //allows you to access other components, like a toke generator class cookieComponent extends Component { public function setBrowserId() { if(!$this->getBrowserId()) { //set a new cookie $guestIDCookie = uniqid('', true); setcookie('guestCookieTest', $guestIDCookie, time() + (86400 * 30), "/"); // 86400 = 1 day } else { //reset the time on existing cookie $guestIDCookie = $_COOKIE['guestCookieTest']; setcookie('guestCookieTest', $guestIDCookie, time() + (86400 * 30), "/"); // 86400 = 1 day } return $guestIDCookie; } public function getBrowserId() { if(!isset($_COOKIE['guestCookieTest']) || $_COOKIE['guestCookieTest'] == '') { return false; } else { return $_COOKIE['guestCookieTest']; } } public function deleteBrowserId() { setcookie('guestCookieTest', '', time() - 3600, "/"); } }
Step 2: Make the Cookie component available to your CakePHP 4 application
In your src\Controller\AppController.php file, add the following to the initialize() function:
public function initialize(): void {
parent::initialize();
$this->loadComponent("cookie");
...
Step 3: Usage examples
Now that the cookieComponent is loaded, you can use it throughout your entire application! In your controller you would use the following:
$browserCookieId = $this->cookie->getBrowserId(); //check for cookie and if none, set it
Delete the cookie using the following:
$this->cookie->deleteBrowserId();