Quick Example of using Google's reCaptcha (v2) in PHP
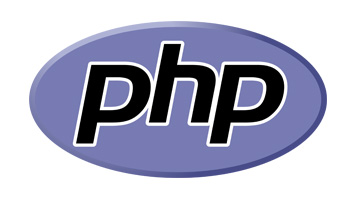
Quick example for PHP in implementing reCaptcha (v2)
Users get the following checkbox; If google isn't sure when checked, they prompt with photo tiles, to identify things like traffic lights, etc.
Step 1: Go to Google and setup your website to get your keys
https://www.google.com/recaptcha/
Step 2: Get your keys from Google
- Go to your admin console from the site above.
- Select your website (from the above step 1)
- Copy your keys as shown below
Step 3: Create your HTML form for posting
- Create the form you wish to use for this plugin.
- In that PAGE (<head>), you will need to call the Google Captcha library.
-
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
-
- Inside the FORM, place the follwing code:
-
<div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div>
-
- Optional: If you want to test to see if user checked the reCaptcha in jQuery:
<script> $('#your-form-id').submit( function() { if($('#g-recaptcha-response').val() == '') { alert('Please check the "I\'m not a robot" reCAPTCHA box.'); return false; } }); </script>
Step 4: Post to the Google API and find success or failure. See the PHP example function below.
Use the following function to check Google's response to the posted-checked results from the visitor.
$gRecaptchaResponse = $_POST['g-recaptcha-response']); $result = checkReCaptcha($gRecaptchaResponse); var_dump($result); function checkReCaptcha($gRecaptchaResponse) { $url = 'https://www.google.com/recaptcha/api/siteverify'; // Post to google $postdata = http_build_query( ["secret"=>"Your_Google_Secret_Key", "response"=>$gRecaptchaResponse, //response-field generated from user-not-robot-click "remoteip"=>$_SERVER['REMOTE_ADDR'] //optional ]); $postsettings = ['http' => [ 'method' => 'POST', 'header' => 'Content-type: application/x-www-form-urlencoded', 'content' => $postdata ] ]; $context = stream_context_create($postsettings); $result = file_get_contents($url, false, $context); $result = json_decode($result); return $result; }