Square Connect with PHP & CURL - Get Customers
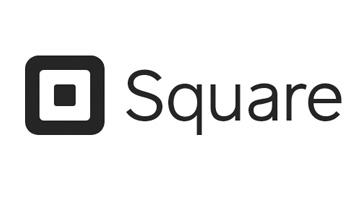
Square Payments API with PHP & CURL - Get Customer List
This example demonstrates getting a customer list from Square, using only PHP & Curl.
* You need an acess token from Square. This example assumes you already have it.
Create new file, for example get-square-customers.php, paste the following and view the list browse to it on your web server.
//set endpoint & access token. //$endpoint_host = 'connect.squareup.com/v2/'; //production $endpoint_host = 'connect.squareupsandbox.com/v2/'; //sandbox $access_token = 'YOUR_APP_ACCESS_TOKEN'; //from Square App Dashboard $idempotency_key = uniqid(); //Set verify_ssl to false for localhost/unsigned SSLs, and true for production $verify_ssl = false; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => $endpoint_host.'customers?cursor=', CURLOPT_SSL_VERIFYHOST => $verify_ssl, CURLOPT_SSL_VERIFYPEER => $verify_ssl, CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 60, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', CURLOPT_HTTPHEADER => array( 'Authorization: Bearer '.$access_token ), )); $response = curl_exec($curl); $err = curl_error($curl); $response = json_decode($response); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { var_dump($response); }
Square API Docs: https://developer.squareup.com/reference/square/customers-api/list-customers