Encrypting & Decrypting With PHP Using OpenSSL
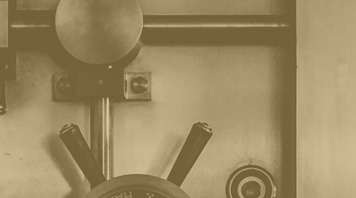
Encrypting & Decrypting With PHP Using OpenSSL
This simple example demonstrates how to encrypt and decrypt a string in php.
Note: This requires the Open SSL extension in your php.ini file. Set, or uncomment the following in your php.ini file: extension=openssl to enable it.
Drop the following into a .php page to test it.
<?php $string_to_encrypt = "This is a string I want to encrypt"; $encryption = new Encryption; echo '<br/>Encrypted Value: ' . $encryption->encrypt($string_to_encrypt); echo '<br/>Decrypted Value: ' . $encryption->decrypt($encryption->encrypt($string_to_encrypt)); class Encryption { private $encrypt_cipher_method = 'aes-256-xts'; private $encrypt_init_vector = '1234567812345678'; // Must be 16 characters long for this usage private $encrypt_key = 'YOURSECRETPASSWORD'; //any length public function encrypt($string_to_encrypt) { $encrypted_value = openssl_encrypt($string_to_encrypt, $this->encrypt_cipher_method, $this->encrypt_key, 0, $this->encrypt_init_vector ); return $encrypted_value; } public function decrypt($encrypted_value) { $decrypted_value = openssl_decrypt($encrypted_value, $this->encrypt_cipher_method, $this->encrypt_key, 0, $this->encrypt_init_vector); return $decrypted_value; } } ?>